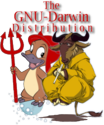
|
Base class/standard interface for objects which require IO support, cloning and reference counting.
Inheritance:
Public Methods-
inline Object()
- Construct an object.
-
Object(const Object&, const CopyOp& copyop=CopyOp::SHALLOW_COPY)
- Copy constructor, optional CopyOp object can be used to control shallow vs deep copying of dynamic data
-
virtual Object* cloneType() const = 0
- Clone the type of an object, with Object* return type.
-
virtual Object* clone(const CopyOp&) const = 0
- Clone the an object, with Object* return type.
-
virtual bool isSameKindAs(const Object*) const
-
virtual const char* libraryName() const = 0
- return the name of the object's library.
-
virtual const char* className() const = 0
- return the name of the object's class type.
-
inline void setDataVariance(DataVariance dv)
- Set the data variance of this object.
-
inline DataVariance getDataVariance() const
- Get the data variance of this object
-
inline void setUserData(Referenced* obj)
- Set user data, data must be subclased from Referenced to allow automatic memory handling.
-
inline Referenced* getUserData()
- Get user data
-
inline const Referenced* getUserData() const
- Get const user data
Public Members-
enum DataVariance
Protected Fields-
DataVariance _dataVariance
-
ref_ptr<Referenced> _userData
Protected Methods-
virtual ~Object()
- Object destructor.
Public Methods-
inline Referenced& operator = (Referenced&)
-
static void setDeleteHandler(DeleteHandler* handler)
-
static DeleteHandler* getDeleteHandler()
-
inline void ref() const
-
inline void unref_nodelete() const
-
inline int referenceCount() const
-
inline void unref() const
Protected Fields-
mutable int _refCount
Documentation
Base class/standard interface for objects which require IO support,
cloning and reference counting.
Based on GOF Composite, Prototype and Template Method patterns.
inline Object()
- Construct an object. Note Object is a pure virtual base class
and therefore cannot be constructed on its own, only derived
classes which override the clone and className methods are
concrete classes and can be constructed.
Object(const Object&, const CopyOp& copyop=CopyOp::SHALLOW_COPY)
- Copy constructor, optional CopyOp object can be used to control
shallow vs deep copying of dynamic data
virtual Object* cloneType() const = 0
- Clone the type of an object, with Object* return type.
Must be defined by derived classes.
virtual Object* clone(const CopyOp&) const = 0
- Clone the an object, with Object* return type.
Must be defined by derived classes.
virtual bool isSameKindAs(const Object*) const
virtual const char* libraryName() const = 0
- return the name of the object's library. Must be defined
by derived classes. The OpenSceneGraph convention the is
that the namspace of a library is the same as the library name.
virtual const char* className() const = 0
- return the name of the object's class type. Must be defined
by derived classes.
enum DataVariance
DYNAMIC
STATIC
inline void setDataVariance(DataVariance dv)
- Set the data variance of this object.
Can be set to either STATIC for values that do not change over the lifetime of the object,
or DYNAMIC for values that vary over the lifetime of the object. The DataVariance value
can be used be routines such as optimzation codes that wish to share static data.
inline DataVariance getDataVariance() const
- Get the data variance of this object
inline void setUserData(Referenced* obj)
-
Set user data, data must be subclased from Referenced to allow
automatic memory handling. If you own data isn't directly
subclassed from Referenced then create and adapter object
which points to your own objects and handles the memory addressing.
inline Referenced* getUserData()
- Get user data
inline const Referenced* getUserData() const
- Get const user data
virtual ~Object()
- Object destructor. Note, is protected so that Objects cannot
be deleted other than by being dereferenced and the reference
count being zero (see osg::Referenced), preventing the deletion
of nodes which are still in use. This also means that
Node's cannot be created on stack i.e Node node will not compile,
forcing all nodes to be created on the heap i.e Node* node
= new Node().
DataVariance _dataVariance
ref_ptr<Referenced> _userData
- Direct child classes:
- TessellationHints
StateSet
StateAttribute
Shape
RefMatrix
PrimitiveSet
NodeCallback
Node
Image
Drawable
ConvexPlanarOccluder
Array
AnimationPath
Alphabetic index HTML hierarchy of classes or Java
This page was generated with the help of DOC++.
|