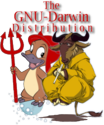
|
with various data-link layer, network layer, routing and transport
layer networking protocols. It has been specifically developed for
undergraduate teaching.">
undergraduate, teaching">
TEXT ="black" LINK="blue" VLINK="purple">
cnet's Timers
A total of 10 software timer queues
are supported to provide a call-back mechanism for user code.
For example,
when a data frame is transmitted a timer is typically created which will
expire sometime after that frame's acknowledgement is expected.
Timers are referenced via unique values termed timers
of datatype CnetTimer.
When a timer expires,
the event handler for the corresponding event
(one of EV_TIMER1..EV_TIMER10)
is invoked with the event and unique timer as parameters.
Timers may be cancelled with CNET_stop_timer
to prevent them expiring
(for example, if the acknowledgement frame arrives before the timer expires).
Timers are automatically cancelled as a result of their handler being invoked.
There are an unlimited number of timers
It is important to understand that an unlimited number of timers may be
started on each queue - there are not just 10 timers.
For example, the following section of code:
void timeouts(CnetEvent ev, CnetTimer timer, CnetData data)
{
int i = (int)data;
(void)printf("tick number %d\n", i);
}
void reboot_node(CnetEvent ev, CnetTimer timer, CnetData data)
{
(void)CNET_set_handler(EV_TIMER3, timeouts, 0);
for(i=1 ; i<=10 ; ++i)
(void)CNET_start_timer(EV_TIMER3, i*1000L, (CnetData)i);
}
|
|
will produce output identical to that from:
void timeouts(CnetEvent ev, CnetTimer timer, CnetData data)
{
int i = (int)data;
printf("tick number %d\n", i);
if(i < 10)
(void)CNET_start_timer(EV_TIMER3, 1000L, (CnetData)i+1);
}
void reboot_node(CnetEvent ev, CnetTimer timer, CnetData data)
{
(void)CNET_set_handler(EV_TIMER3, timeouts, 0);
(void)CNET_start_timer(EV_TIMER3, 1000L, (CnetData)1);
}
|
|
-
CnetTimer
CNET_start_timer(CnetEvent ev, long msec, CnetData data)
-
Requests that a new timer be created which will expire in the
indicated number of milliseconds.
One of the 10 timer queues
may be requested by passing one of the event types
EV_TIMER1..EV_TIMER10.
A unique timer is returned to distinguish this newly created
timer from all others.
This timer should later be used in subsequent
calls to CNET_stop_timer().
If a new timer cannot be created, NULLTIMER will be returned.
Possible errors: ER_BADARG.
-
int
CNET_stop_timer(CnetTimer timer)
-
Requests that the indicated timer be cancelled (before it has expired).
Possible errors: ER_BADTIMER.
-
int
CNET_timer_data(CnetTimer timer, CnetData *data)
-
Allows the CnetData value for the indicated CnetTimer value
to be recovered. The timer is not cancelled.
Possible errors: ER_BADTIMER, ER_BADARG.
|